简介
其实要实现拖拽的控件是非常简单的事情,和让控件支持点击一样简单!
我们只需要实现onDragEvent()
dispatchDragEvent()
setOnDragListener()
即可
是不是和触摸事件的回调差不多呢?
(onTouchEvent()
dispatchTouchEvent()
setOnTouchListener()
)
我们可以通过View.startDarg(ClipData data, DragShadowBuilder shadowBuilder, Object myLocalState, int flags)
来开始拖拽
- 这里需要我们传入一个 DragShadowBuilder 对象,DragShadow 就是拖拽阴影,会在你拖动的时候显示出来
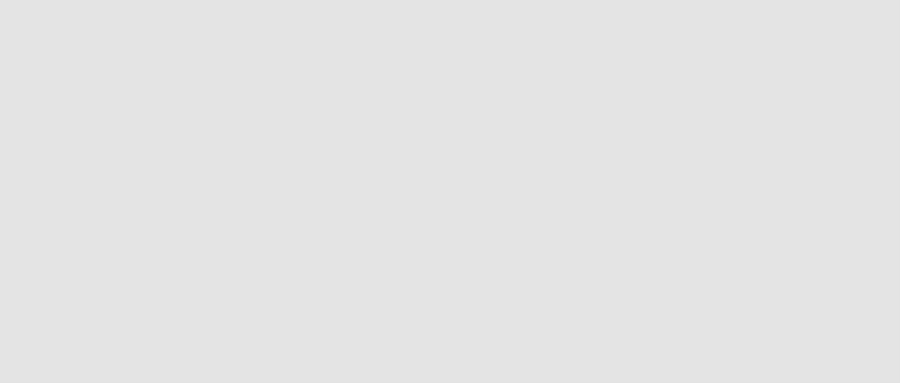
实现
MainActivity.java1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112
| package cn.gavinliu.drag;
import android.app.Activity; import android.content.ClipData; import android.graphics.Color; import android.os.Bundle; import android.util.Log; import android.view.DragEvent; import android.view.View; import android.view.View.DragShadowBuilder; import android.view.View.OnDragListener; import android.view.View.OnLongClickListener; import android.widget.ImageView;
public class MainActivity extends Activity {
private String TAG = "DragDemo";
private ImageView image; private ImageView image2;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
image = (ImageView) findViewById(R.id.image); image2 = (ImageView) findViewById(R.id.image2);
image.setOnLongClickListener(new OnLongClickListener() {
@Override public boolean onLongClick(View v) { DragShadowBuilder builder = new DragShadowBuilder(v); ClipData data = ClipData.newPlainText("dot", "Dot : " + v.toString()); v.startDrag(data, builder, v, 0); return true; } });
image.setOnDragListener(new OnDragListener() {
@Override public boolean onDrag(View v, DragEvent event) { final int action = event.getAction(); switch (action) { case DragEvent.ACTION_DRAG_STARTED: image.setVisibility(View.INVISIBLE); Log.d(TAG, "image1 ACTION_DRAG_STARTED"); break; case DragEvent.ACTION_DRAG_ENDED: image.setVisibility(View.VISIBLE); Log.d(TAG, "image1 ACTION_DRAG_ENDED"); break; case DragEvent.ACTION_DRAG_EXITED: Log.d(TAG, "image1 ACTION_DRAG_EXITED"); break; case DragEvent.ACTION_DRAG_LOCATION: Log.d(TAG, "image1 ACTION_DRAG_LOCATION"); break; case DragEvent.ACTION_DRAG_ENTERED: Log.d(TAG, "image1 ACTION_DRAG_ENTERED"); break; case DragEvent.ACTION_DROP: Log.d(TAG, "image1 ACTION_DROP"); break; } return true; } });
image2.setOnDragListener(new OnDragListener() {
@Override public boolean onDrag(View v, DragEvent event) { final int action = event.getAction(); switch (action) { case DragEvent.ACTION_DRAG_STARTED: Log.d(TAG, "image2 ACTION_DRAG_STARTED"); break; case DragEvent.ACTION_DRAG_ENDED: image2.setBackgroundColor(Color.TRANSPARENT); Log.d(TAG, "image2 ACTION_DRAG_ENDED"); break; case DragEvent.ACTION_DRAG_EXITED: image2.setBackgroundColor(Color.TRANSPARENT); Log.d(TAG, "image2 ACTION_DRAG_EXITED"); break; case DragEvent.ACTION_DRAG_ENTERED: image2.setBackgroundColor(Color.BLUE); Log.d(TAG, "image2 ACTION_DRAG_ENTERED"); break; case DragEvent.ACTION_DRAG_LOCATION: Log.d(TAG, "image2 ACTION_DRAG_LOCATION"); case DragEvent.ACTION_DROP: Log.d(TAG, "image2 ACTION_DROP"); } return true; } }); }
}
|
activity_main.xml1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" >
<ImageView android:id="@+id/image" android:layout_width="100dp" android:layout_height="100dp" android:src="@drawable/ic_launcher" />
<ImageView android:id="@+id/image2" android:layout_width="100dp" android:layout_height="100dp" android:layout_alignParentRight="true" android:layout_below="@id/image" android:src="@drawable/ic_launcher" />
</RelativeLayout>
|
详细描述请参见官方文档:http://developer.android.com/guide/topics/ui/drag-drop.html