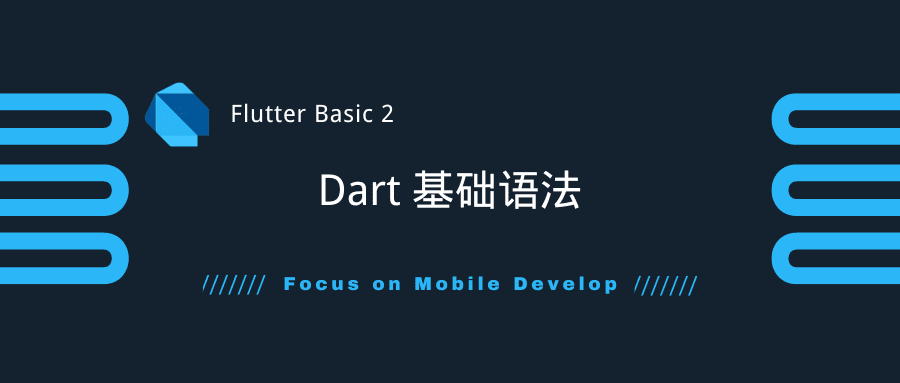
Flutter 基础 2 | Dart 基础语法
本文主要是对 Dart 官网的 Language tour
的学习笔记,完整内容详细阅读官方文档
https://dart.dev/guides/language/language-tour
重要的概念
- 所有能够使用变量引用的东西都是对象,每个对象都是一个类的实例。甚至数字、函数、null 都是对象,都继承至 Object
- 尽管 Dart 是强类型的,但类型注释可以不声明,因为 Dart 有类型检查,但是为了代码的静态分析,你应该用完整的类型注释声明变量。
- Dart 支持泛型,
List<int>
表示一个整数列表,List<dynamic>
表示任意类型列表 - 不同于 Java,Dart 没有
public
,protected
, andprivate
关键字,如果要表示私有的,以 _ 开头命名。
变量
- 使用
var
或变量类型
来声明变量:
1 | var age = 14; |
因为
Dart
能够用变量引用的东西都是对象,所以int
的默认值同样是null
使用
final
或const
来声明常量:
const 表示编译时就能确定的常量;
final 表示运行时确定的常量
类型
Dart 的内置类型如下:
- numbers
int
64bitsdouble
64bits
- strings
String
UTF-16 字符串
- booleans
bool
布尔值
- lists
List
和数组一样
- sets
Set
唯一的无序集合
- maps
Map
键值对
- runes
Runes
另一种字符串,UTF-32,emoji
- symbols
Symbol
是一种编译时的常量,不会被混淆,通常和混淆dart:mirrors
一起用
函数
函数声明和大多数程序都一样
1 | bool isNoble(int atomicNumber) { |
对于返回值是 void 的函数,void 可以省略。
可选参数
参数命名
用下面这种方式命名的参数,调用时可以不填,填的话调用时必须指定参数名
1 | void hello({int y, bool x}) { |
当然你依然可以指定命名参数为必填,通过 package:meta/meta.dart
中的 @required
来修饰必填参数
1 | import 'package:meta/meta.dart'; |
可选参数
1 | String say(String from, String msg, [String device]) { |
参数默认值
1 | void enableFlags({bool bold = false, bool hidden = false}) {...} |
1 | String say(String from, String msg,[String device = 'carrier pigeon', String mood]) {...} |
闭包
操作符
和大多数编程语言一样,下面举一些不一样的:
类型检查操作符
as
类型转换is
is!
类型判断
条件运算符
a ?? b
如果 a == null 则返回 b
流程控制
和大多数编程语言一样
if
andelse
for
loopswhile
anddo-while
loopsbreak
andcontinue
switch
andcase
assert
断言
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 Gavin Liu - 代码人生与科技生活
评论