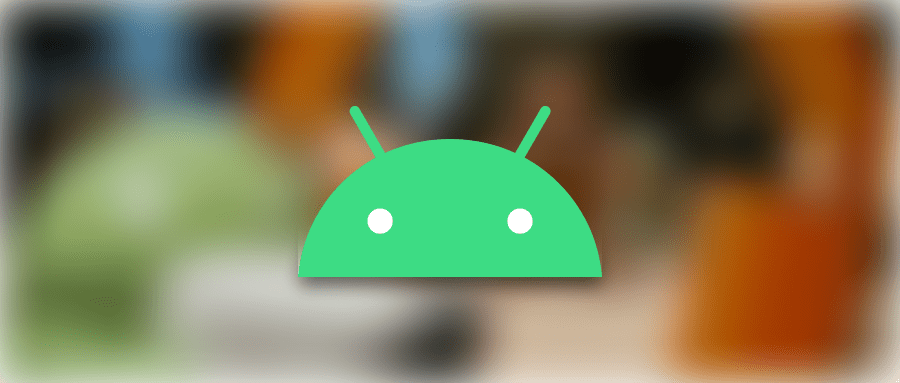
Android | 实用代码片段(3)
- 在 View Layout 完成后获取 控件大小
1 | final TextView tv = (TextView) findViewById(R.id.myTextView); |
- 关键帧插值器
1 | public static float calculateValue(float[] values, float time, float def) { |
- 给图片叠加渐变
1 | private void addShadow(Bitmap bitmap, int color) { |
- ListView 或者 GridView 去除滑动特性 (即固定高度)
1 |
|
- 获取系统长按时间,自定义 View 会用到
1 | getSystemLongPressTime |
- 判断设备是否有网
1 | public static boolean isOnline(Context context) { |
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 Gavin Liu - 代码人生与科技生活
评论